QX82 is a tiny Javascript engine that lets you create games and experiences inspired by the look and feel of a retro 80s computer. It's not an emulator or a fantasy console: it's just a Javascript library.
It's open source. You can download it from the GitHub repo.
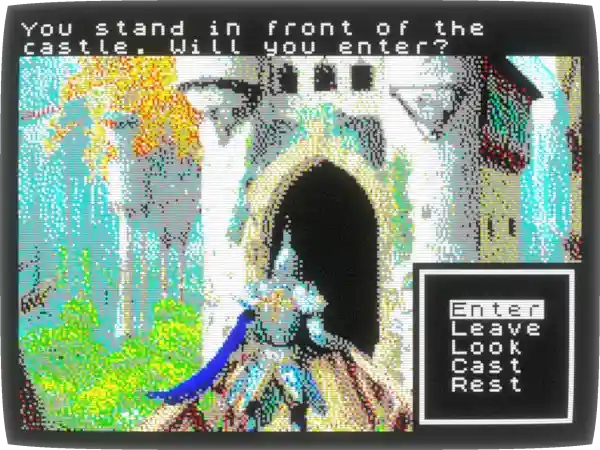
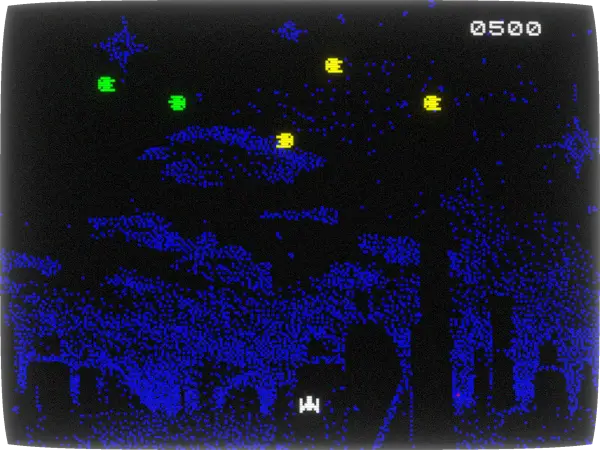

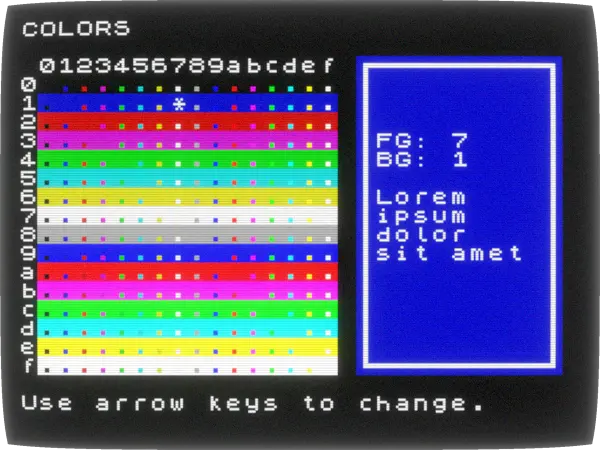
Although this engine tries to recreate the feel of retro computers, you are not limited to the functionality of old computers: this is just Javascript and you can do whatever you want with no artificial restrictions.
It's open-source and MIT-licensed, so you can freely see how it works and make modifications to it as you need.
Want to see it in action? Check out the demos (use arrow keys to move):
This engine was developed by Bruno Oliveira. I'm @btco_code on Twitter and on Mastodon. If you want to write me an email, my email address is my first name followed by "tc" at gmail.com.
Note about mobile support: This engine was mostly designed for desktop, but on mobile devices it shows a virtual joystick with a DPAD and 2 buttons. However, mobile support is currently very experimental and quite buggy, I'll try to fix it soon!
What does the API look like?
It's a straightforward immediate mode API inspired by BASIC:
// Clear screen (7 = white, 1 = blue). qx.color(7, 1); qx.cls(); // Print hello at pos (1,1). qx.locate(1, 1); qx.print("Hello world!\n\n"); // Ask the user's name. qx.print("What is your name? "); // Wait for user to type: const name = await qxa.readline(); // Print back the name in yellow (14). qx.color(14); qx.print(`\n\nHi, {name}.`);
There's also a more traditional frame-based API where you get a callback to draw every frame, which works best for action games that run continuously without blocking for user input.
Graphics
Like in the old times, a lot of graphics is accomplished via characters. You can define the graphics for each character code, so you can use this for the player, enemies, projectiles, fancy borders, or anything else you want.
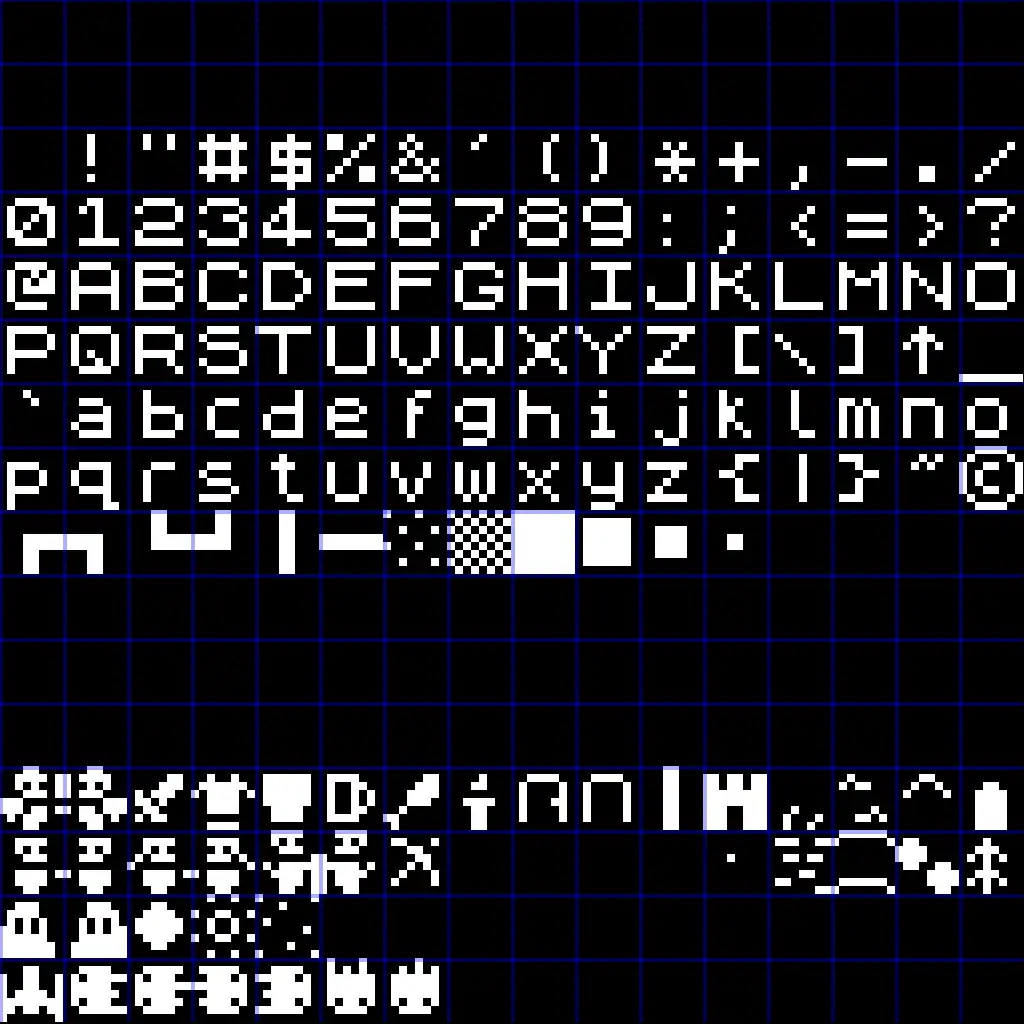
You can of course change this font file to draw anything you want. Then you can use the character code (these are numbered from 0x00 to 0xFF) to draw:
// Set color (yellow over blue). qx.color(14, 1); // Draw hero (char code 0xc0). qx.locate(5, 5); qx.print("\uc0")
And if you don't want to be constrained by text-mode rows and columns, you can draw directly using pixel coordinates too:
// Draw hero at pixel pos (50, 50). qx.spr(0xc0, 50, 50);
Ready to create your game? Continue to the How-To section.